This lesson introduces the basic components of Python programming and examines what they are, what their grammar is, what they do, and so on. There are also many instances of the standard Python scripting method recommended to Python programmers by the PEP 8 document ; Adherence to these principles contributes to the uniformity of Python community codes.
Syntax ( Syntax or syntax) is a set of rules that defines how to program in a language specified; For example, how a text is written to be interpreted by a Python interpreter or a string object depends on the syntax defined in Python, and an exception will be reported if the interpreter fails to conform to any of the defined rules. Python syntax is not limited to this lesson, and you will see many other things, such as how to define different objects, in future lessons.
✔ Level: Introductory
Lines
The Python interpreter, as well as the user, sees the code inside each module in the form of a number of lines; Rows physical (Physical Lines) and logical (Logical Lines). The physical lines are actually the lines that are numbered by the text editors and are easily recognizable by the user, but the logical lines are the interpreter’s perception of the program components; Each logical line represents a Python statement. For example, consider the command print
in Python 2x:
msg = "Welcome!" print msg
In the example code above: the first line shows an Assign command; This command msg
attributes its right value to the variable . You are more or less familiar with the second line command. This command displays the value of the received variable on the output. There are two commands here, two logical lines, each implemented in the form of a physical line.
Although it is suggested that each physical line always contain only one logical line, a physical line can also contain several logical lines:
msg = "Welcome!"; print msg
|
In this case, the logical lines (or commands) must be separated by a character (Semicolon).
Sometimes it is better to implement a logical line in the form of several physical lines for more readability:
msg = "Python Programming \ Language." # This is a message. print msg
In the example of the above code: the first two physical lines are seen as only one logical line from the commentator’s point of view. In Python, a character \
(Backslash) is used to break command in several physical lines . Note, however, that it \
can not be used to break the comment line, nor can any explanation be inserted afterwards.
tip
[ PEP 8 ]: The length of each physical line should not exceed 79 characters. For long texts such as Comment and Docstring, the length of each physical line must be a maximum of 72 characters.
It is better to break long sentences for more readability. Commands that contain, and are, can be written without fail and in the form of several physical lines:{ }
[ ]
( )
\
month_names = ['Januari', 'Februari', 'Maart', # These are the 'April', 'Mei', 'Juni', # Dutch names 'Juli', 'Augustus', 'September', # for the months 'Oktober', 'November', 'December'] # of the year
In this case, despite the use \
, the explanation can be added after breaking the lines.
” Blank lines » (Blank lines): a line that contains only empty space (Space or Tab) be, by the bytecode interpreter is ignored and not translated. These lines can be used to make the code more readable – like the third line in the example code below:
def power(a, b): return a ** b print power(2, 3)
Documentation
Although the Python language design is based on high code readability, program “documentation” means using features such as code explanations to better understand and read program code for future reference by developers and others who want to develop. It will also be very useful to be active or use it. In this section, we will examine the two possibilities of inserting Comment and Docstring to document the program.
Description
A ” comment ” in Python # begins with a character and ends with a physical line. Descriptions, like blank lines, are ignored by the interpreter and are not translated into bytecodes.
The purpose of writing a description among codes is to describe the logic of a piece of code and why the code is written and what it does. Sometimes the explanation feature (which is ignored by the interpreter) is used to disable the code. Explanation plays a big role in the readability of the code and its regular use is recommended.
The explanation in Python is defined as a single line only, and to insert descriptions with more than one physical line, it must be noted that each line must #begin separately.
tip
[ PEP 8 ]: Description text with a space after it #starts. In multi-line explanations, use a line without description (blank line that #begins with) to separate paragraphs . When inserting an explanation in the same lines of the command, the explanation should be at least two spaces away from the end of the command.
# A comment, this is so you can read your program later. # Anything after the # is ignored by python. print "I could have code like this." # and the comment after is ignored # You can also use a comment to "disable" or comment out a piece of code: # print "This won't run." print "This will run."
Docstring
Next to “explanation”; “Docstring” is another feature in Python for more explanation of application code. The Docstring text begins and ends with three quotation marks ( """
or '''
) [ PEP 257 ] and is usually used as the first command in a module, class, function, and method, in which case the Docstring is not ignored by the interpreter at runtime. It can also __doc__
be achieved by using the adjective :
def complex(real=0.0, imag=0.0):
"""Form a complex number.
Keyword arguments:
real -- the real part (default 0.0)
imag -- the imaginary part (default 0.0)
"""
>>> complex.__doc__ 'Form a complex number.\n\n Keyword arguments:\n real -- the real part (default 0.0)\n imag -- the imaginary part (default 0.0)\n ' >>> print(complex.__doc__) Form a complex number. Keyword arguments: real -- the real part (default 0.0) imag -- the imaginary part (default 0.0) >>>
consideration
n\
Indicates the end of the current line and goes to the next line –n\
you should
The addressee of the “description” text in the code is the users who develop that code, while the main addressee of the Docstring is the users who use the code, so the Docstring must explain how to use the code (specifically: module, function , Class and method).
Docstring should be inserted as the first command, and this point is considered for a module if there are lines of interpreter execution and coding specified below:
#!/usr/bin/env python #-*- coding: utf-8 -*- """ Module docstring. """ import [...] [...]
Packages can also have Docstring; For this purpose, Docstring must init__.py__
be written inside the module.
tip
Docstrings can be used anywhere in program code as an alternative to multi-line descriptions, in which case the interpreter is ignored and can no longer be accessed.
Indentation
Blocking in Python is defined by the ” Indentation ” of the lines; This is done in brackets in languages like C by braces. Indentation is the amount of free space (Spaces and Tabs) of each command from the beginning of its physical line. The important point is that all the commands in a block must be indented at some distance from their header:{ }
// C if (x > y) { x = 1; y = 2; }
# Python if x > y: x = 1 y = 2
In the image below, pay attention to the way the blocks are indented towards your header:

tip
[ PEP 8 ]: Use the Space key to create an indentation over the Tab key – use four Space keys for each indentation.
A common way to create indentations is to use the Space key, and never try to use the Sapce and Tab keys in combination, although it is not possible to use a combination of these two keys in the 3x Python version! If you want to use the Tab key, you have to create all the indentations of your program evenly using it.
There is no compulsion to indent the part of the command that is broken into other physical lines, but for higher readability, it is better to write these parts with a little more indentation than the commands of the current block body:
def long_function_name( var_one, var_two, var_three, var_four): print(var_one)
In commands such as the following, creating an argument alignment is also appropriate:
foo = long_function_name(var_one, var_two, var_three, var_four)
Command
A ” statement ” is a unit of code that contains keywords, is executed, and does something. There are two types of commands in Python:
Simple commands ( Simple Statements ): statements that are implemented in a logical row. Such as command import
, command pass
, assignment command, function call, etc.
Orders compound ( Compound Statements ): a group of commands that can be a part (eg command def
– defined function) or part (such as the condition command if
/ elif
/ else
) are; Each clause also includes a header and a suite. Each header starts with a keyword and ends with a :
(Colon). The body is written after the head and observing the indentation level more than it:
def binary_search(seq, key): lo = 0 hi = len(seq) - 1 while hi >= lo: mid = lo + (hi - lo) // 2 if seq[mid] < key: lo = mid + 1 elif seq[mid] > key: hi = mid - 1 else: return mid return False
ID
” ID » ( Identifier ) is a name symbolic to determine user preferences and to identify (identify) variables, functions, classes, modules or other Python objects from each other is used. There are some things to consider when choosing an ID in Python:
- Start with just one uppercase or lowercase letter of the English alphabet (
A..Z
ora..z
) or character_
(Underscore). - The following can be given any or more letters of the English alphabet (uppercase and lowercase), characters
_
and numbers (9..0
) – in any order. - There is no limit to the length of the ID and it can be from one to any number of characters.
With a more professional look, the lexical structure of the identifier is expressed below [ Python documents ]:
identifier ::= (letter|"_") (letter | digit | "_")* letter ::= lowercase | uppercase lowercase ::= "a"..."z" uppercase ::= "A"..."Z" digit ::= "0"..."9"
consideration
In regex definitions: parentheses () are used for grouping. Symbol | It means or is and is used to separate two different expressions. The symbol * means zero repetition or more. [A separate course will be dedicated to regex]
tip
-
The use of special characters, such as
.
,!
,@
,#
,$
,%
and is not permitted. -
The use of “Space” is not allowed.
-
The use of “hyphen” (character)
-
to separate words in the module name is allowed but not recommended.
For example – some correct IDs:
a _p __var MyClass get_length getLength var2 var_2 S01E16
For example – some incorrect IDs:
me@mail get.length 2_var 6 $var 4pi
tip
As we learn from Lesson 1, Python is a case-sensitive language, and its interpreter distinguishes between lowercase and uppercase, such as a and A.
For example, all CaR, cAR, CAr, caR, cAr, Car, car, and CAR identifiers are evaluated differently.
Python uses a technique called Name Mangling . By this technique, and only by selecting the identifiers, they are given a special role:
- Private ID Module: If an ID
_
starts (not ends) with a character, it is evaluated by the Python interpreter in this role. Such as:name_
(and not:_name_
or_name
) - Private Class Id: If an ID with two characters
_
begins (rather than ends), it is evaluated by the Python interpreter in this role. Such as:name__
(and not:__name__
or__name
)
Apart from this, in Python there are certain attributes and methods that are predefined and have a specific meaning for the interpreter. The identifier of these attributes and methods _
begins and ends with two characters ; Just like attributes __class__
and __doc__
previously used.
Therefore _
, we must be sufficiently aware when using the character in the ID (especially at the beginning). [The issues will be addressed in the future.]
tip
[ PEP 8 ]: The standard way to select an identifier for a class, function, method, or variable is as follows:
-
Classes are named in PascalCase – meaning that only the first letter of each word is capitalized and the words are spaced apart. Such as: AnimalClass, Animal.
-
The name chosen for a function and method should also contain only lowercase letters and be used to separate words
_
. Such as: bubble_sort, binary_search, etc. Of course, we can also use the camelCase method (like PascalCase, with the difference that the first letter of the first word must also be a lowercase letter). Such as: bubbleSort, binarySearch and … -
The letter of the variables should only contain lowercase letters whose words
_
are separated by each other. Such as: body_color, color and …
For single-character identifiers, be sure to avoid the letters l (lowercase) and I (upper case), as these two letters are similar in some fonts, as well as the letter O (uppercase), which can be zero.
Keywords
The final point of the argument is: can an ID of one of the ” keywords » (keywords) chose Python. Keywords are actually identifiers that are pre-defined for the Python interpreter and have a specific meaning for it. The list of words in Python is as follows:
>>> # Python 3.x >>> help("keywords") Here is a list of the Python keywords. Enter any keyword to get more help. False def if raise None del import return True elif in try and else is while as except lambda with assert finally nonlocal yield break for not class from or continue global pass
>>> # Python 2.x >>> help("keywords") Here is a list of the Python keywords. Enter any keyword to get more help. and elif if print as else import raise assert except in return break exec is try class finally lambda while continue for not with def from or yield del global pass
There is a module in the standard Python library called keyword [Python Documents ]:
>>> # Python 3.x >>> import keyword >>> keyword.iskeyword(a) False >>> keyword.iskeyword("def") True >>> keyword.kwlist ['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
consideration
The function
()iskeyword
checks whether the received argument is one of the keywords; Returns the value if confirmedTrue
.kwlist
Also, a list object contains all the keywords.
You may be curious to know the number of Python keywords; You do not need to count manually for this purpose !:
>>> # Python 3.x >>> import keyword >>> len(keyword.kwlist) 33 >>> # Python 2.x >>> import keyword >>> len(keyword.kwlist) 31
consideration
The function
()len
returns the number of members of an object [ Python documents ].
Some tips:
- Python 3x: only keywords
True
,False
andNone
capitalized start. - In Python 2x: Keywords
True
,False
andNone
not defined. - In Python 3x: Because the print has become a function, the keyword
print
is not defined.
tip
[ PEP 8 ]: If you want to select an identifier similar to one of the keywords; You can do this by using one
_
at the end of the word. As:_def
Variable
A ” variable ” in most programming languages, such as C, indicates a location in memory in which a value is stored. Consider the following three commands, for example:
int a = 1; a = 2; int b = a;
In the example code above: the command; int a = 1 states that a memory location called a should be considered for storing integers and the value 1 should be there; From now on, the variable a represents this point in memory (just like a box) that now contains the value 1 (Figure below – one). Then the command; a = 2 causes the previous value of the variable a to be removed (out of the box) and the new value of 2 to be placed in it (bottom figure – two). Note that in this category of languages, the type is specified by the variable, and attempting to insert a data type other than int into the variable a (such as 3.7 or “string”) causes a compilation error. Third command: ; int b = a first creates a new location in memory called b of the same integer type and then copies the value inside the variable a into it; There are now two places to store the int data type in memory, both of which contain a value of 2 (Figure 3 below).
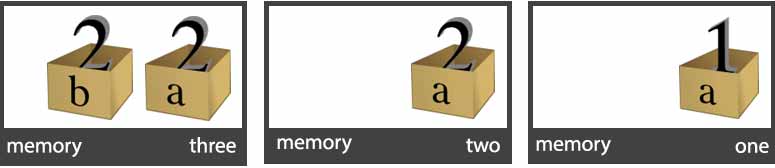
But in Python:
A variable is nothing more than a name that refers to (or refers to) a specific object in memory. Defining a variable in Python is very simple and can only be done by assigning an object to a name. The symbol =
is the Assignment Operator in Python. In defining the Python variable, unlike what we saw in C, ;int a
، there is no need to specify a type for it because type is determined by the object and a variable can refer to objects of different types during runtime. Consider the following three commands, for example:
a = 1
a = 2
b = a
The interpreter performs the following three steps upon reaching the command :a = 1
- Creates an object of type integers and values
1
somewhere in memory. Why integers? The type is determined by the object and the1
number is correct! - Creates the variable
a
(or the same name) elsewhere in the memory (if it has not already been created). - Establishes a link from the variable
a
to the object1
. This link is called “Reference” which is implemented as a pointer in memory.
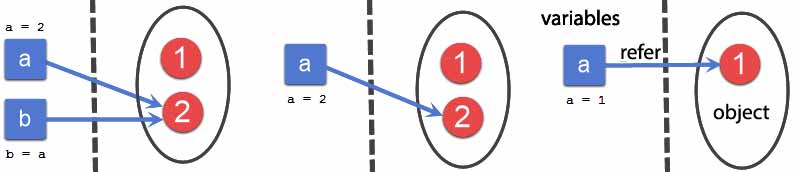
Attribute another object (which can be of any other type) to an existing variable; Deletes the previous reference to the new object. The command a = 2 creates object 2, deletes the reference of variable a to object 1, and creates a new reference from variable a to object 2. Each variable is named to refer to an object; The command b = a also says: Create a new variable named b and have the same reference object as the variable a.
But now that 1
there is no reference to the object, what happens to it?
Each object contains a ” counter-reference » (Reference Counter) as well; In this way, it shows the number of references to that object at each moment, and with each new reference to the object, one unit is added to it, and by deleting each reference, one unit decreases. If its value reaches zero, the object is cleared by the ” Garbage Collection ” technique and the amount of memory consumed by the object is released. The function ()getrefcount
inside the module sys
can be used to view the number of references to an object [ Python Documents ].
Of course, the Python interpreter does not delete integers and small strings from memory after the reference counter value reaches zero. The purpose is to reduce the cost of creating these objects for future use. Therefore, in answer to the above question, it should be said that: the object 1
remains in memory.
>>> import sys >>> a = 1 >>> sys.getrefcount(1) 760 >>> a = 2 >>> sys.getrefcount(1) 759 >>> sys.getrefcount(2) 96 >>> b = a >>> sys.getrefcount(2) 97
1
and 2
out than expected, indicating that the Python interpreter runs in the background there are also other references to these objects.Multiple Assignment
It is possible to create multiple variables or multiple assignments in Python at the same time – you can create multiple variables, all of which refer to the same object:
>>> a = b = c = "python" >>> a 'python' >>> b 'python' >>> c 'python'
To assign different objects we must use a comma and only one assignment operator ( =
) – note that the number of elements on both sides of the assignment operator must be equal:
>>> a, b, c, d = 1, 4.5, "python", 2
>>> a
1
>>> b
4.5
>>> c
'python'
>>> d
2
One of the uses of multiple assignments is that two variable objects can be easily swapped with just one command line:
>>> a = 1
>>> b = 2
>>> a, b = b, a
>>> a
2
>>> b
1
Fixed
” Constant ” is a variable whose value is always constant and after the definition, it is no longer possible to change its value. For example, a constant in Java is defined as follows – after the following command, any attempt to change the constant value HOURS
will encounter an error:
final int HOURS = 24;
|
In Python, there is no possibility of a fixed definition!
In addition to the possibility of creating constants, there are some other things that are overlooked in Python. In fact, Python assumes that its users are smart people who can handle problems; “I trust my users, so I don’t have to do everything for them, a programmer has to be able to take risks,” he says.
But to create a constant, you can define your desired variables in a separate module and import
access the variables you want with it wherever necessary :
# File: constant.py # Path: /home/kazem/Documents/MyModule HOURS = 24
>>> import sys >>> sys.path.append('/home/kazem/Documents/MyModule') >>> import constant >>> constant.HOURS 24
Of course, if the immutability of the variables is of particular importance to you, you can create a module containing the following code [ Source ]:
# File: constant.py # Path: /home/kazem/Documents/MyModule class _const: class ConstError(TypeError): pass def __setattr__(self, name, value): if name in self.__dict__: raise self.ConstError("Can't rebind const(%s)" % name) self.__dict__[name] = value import sys sys.modules[__name__] = _const()
>>> import sys >>> sys.path.append('/home/kazem/Documents/MyModule') >>> import constant >>> constant.HOURS = 24 >>> constant.HOURS 24 >>> constant.HOURS = 23 Traceback (most recent call last): File "<stdin>", line 1, in <module>; File "/home/kazem/Documents/MyModule/constant.py", line 10, in __setattr__ raise self.ConstError("Can't rebind const(%s)" % name) constant.ConstError: Can't rebind const(HOURS) >>> constant.HOURS 24 >>>
Understanding class code const_
requires studying class lessons and Exceptions. But for a brief explanation in this lesson, it should be said that:
The Python interpreter uses a structure similar to {…, name: value}, known as a dictionary, to know which name refers to which value or object; The attributes of each object and their value are also maintained by such a structure that you can use the special attribute __dict__ to view this dictionary, which is actually the same list of attributes of each object with their value. The __setattr__ [Python Documents] method is a special method – these methods allow us to define specific behaviors for specific occasions – __setattr__ is automatically called whenever a value is assigned to one of the object attributes of a class, and its function Store attributes and their value in this dictionary.
Here the behavior of the method is __setattr__
slightly modified to be checked (line 9) if the desired attribute does not already exist (ie: no value has already been assigned to it to be in the list; definition Remember the variable) will be added to the list of object attributes along with the value (line 11); Otherwise, an error will be reported that will stop the execution of the method and as a result will prevent a new entry in the list that changes the value of the desired attribute (line 10).
Modules are also treated as objects in Python, so the Python interpreter must know which module name belongs to which object; sys.modules
A dictionary contains all the modules that are loaded at this point in the program. Refers [__sys.modules[__name
to a member of this dictionary by name __name__
. We know that it __name__
indicates the name of the current module; So the expression [__sys.modules[__name
represents the name that refers to the object of the constant.py module. The line 14 command removes the reference to the module and instead assigns it to an object in the class const_
, which removes the module object from memory (because there is no reference to it anymore). On the other hand, we know that with import
each module, all its contents are executed; Withimport
The constant.py module and after executing its codes, especially line 14, as mentioned, the relevant module is deleted, but its codes still remain in the bytecode. So then we import
can easily use the module name, which now refers to an object in the class const_
, to create attributes that are our constants. [All of these concepts will be fully explored in the future]
tip
[ PEP 8 ]: Use only uppercase letters for naming constants and also for separating words
ـ
. Such as: MAX_OVERFLOW, TOTAL and …
Operators
” Operator ” is a symbol that performs a specific action on objects; Like the assignment operator =
discussed earlier. Objects on which the operator performs an action are also called ” operands “. There are different types of operators, which we will examine below.
1. Arithmetic Operators:+
-
*
**
/
//
%
-
+
Addition: Adds the amount of operands on both sides together.2 + 1
Result: 3 -
-
Subtraction: Subtracts the value of the right operand from the value of the left operand:4 - 7
Result: 3 -
*
Multiplication: Multiplies the amount of operands on both sides:2 * 5
Result: 10 -
**
Power (Exponent): Increases the value of the left operand to the power of the value of the right operand.3 ** 2
Result: 8 -
/
Division: Divides the value of the left operand by the value of its right operand and returns the outside of the part:
>>> # Python 3.x >>> # Python 2.x >>> 7 / 3 >>> 7 / 3 2.3333333333333335 2 >>> 12 / 3 >>> 12 / 3 4.0 4 >>> 6.0 / 2 >>> 6.0 / 2 3.0 3.0
As seen in the sample code above; In 3x versions, the result of each division is always calculated as a decimal number, but in 2x versions, the result of dividing two integers is calculated as an integer in the same way and the decimal value (if any) is omitted. This feature can be added to 2x versions below [ Python Documents ]:
>>> # Python 2.x
>>> from __future__ import division
>>> 7 / 3
2.3333333333333335
>>> 12 / 3
4.0
-
//
Floor Division: Divides the value of the left operand by the value of its right operand and returns the outside by removing the decimal value (if any). The result of this operator is calculated as an integer for integers, note the results of the sample code below:
>>> # Python 3.x >>> # Python 2.x >>> 7 // 3 >>> 7 // 3 2 2 >>> 12 // 3 >>> 12 // 3 4 4 >>> 6.0 // 2 >>> 6.0 // 2 3.0 3.0 >>> 7.0 // 3 >>> 7.0 // 3 2.0 2.0
-
%
Residual (Modulus): Divides the value of the left operand by the value of its right operand and returns the remainder. Result: 13 % 7
2. Comparison Operators:==
=!
<>
<
>
=<
=>
==
Equal: Returns if the values of the operands on both sides are equalTrue
. : False1 == 3
=!
Not Equal: Returns if the values of the operands on both sides are not equalTrue
. : True1 =! 3
<>
Not Equal: It works the same=!
but can only be used in the 2x version of Python. : True1 <> 3
<
Greater Than: Returns if the value of the left operand is greater than the value of the right operandTrue
. : False5 < 3
>
Less Than (Less Than): Returns if the value of the left operand is less than the value of the right operandTrue
. : True5 > 3
=<
Greater Than or Equal: Returns if the value of the left operand is equal to or greater than the value of the right operandTrue
. : True5 =< 7
=>
Less Than or Equal: Returns if the value of the left operand is equal to or less than the value of the right operandTrue
. : False5 => 7
3. Assignment Operators:
-
=
Attributes the right operand to its left operand. If a computational expression is on the right, it attributes the result to the operand on the left:>>> a = 3 >>> b = 2 >>> c = a + b >>> c 5
-
=+
=-
=*
=**
=/
=//
=%
Combined operators (arithmetic assignment): These operators first perform the arithmetic operator operation on the value of the operands on both sides and then attribute the result to the left operand:>>> a += b >>> a 5
>>> a -= b >>> a 1
>>> a *= b >>> a 6
>>> a **= b >>> a 9
>>> # Python 3.x >>> # Python 2.x >>> a /= b >>> a /= b >>> a >>> a 1.5 1
4. Bitwise Operators:&
|
^
~
>>
<<
These operators perform a specific operation on each of the operative bits (s). In Python, to use these operators, it is not necessary to convert numbers to base two (binary), but here we have used binary numbers to better see how they work. In Python, two base numbers must always 0b
start with one :
>>> a = 0b0011
>>> a
3
>>> b = 0b0010
>>> b
2
The function ()bin
can be used to obtain the binary value of a decimal number; Note, however, that this function returns a binary value in text format (String type) – Another point is that in Python interactive mode, entering binary numbers gives a decimal output:
>>> bin(10)
'0b1010'
>>> type(bin(10))
<class 'str'>
>>> 0b1010
10
-
&
: AND is equivalent to a bit – only the output bits1
will be that both bits correspond to its operands1
:>>> a & b 0000 0011 2 0000 0010 (AND) ----------- 0000 0010 = 2
-
|
: OR is equivalent to a bit – only the output bits0
will be that both bits correspond to its operands0
:>>> a | b 0000 0011 3 0000 0010 (OR) ----------- 0000 0011 = 3
-
^
: XOR is equivalent to a bit – only the output bits1
will be if both corresponding bits of its operands are opposite each other:>>> a ^ b 0000 0011 1 0000 0010 (XOR) ----------- 0000 0001 = 1
-
~
Is NOT equivalent to single-bit operands – each bit of its operand converts from0
to1
and vice versa:>>> ~ a 0000 0011 -4 (NOT) ----------- 1111 1100
One way to display binary notation numbers is to invert the bits (0 to 1 and 1 to 0), called the ” One’s Complement ” display of binary numbers. But the Python interpreter by default evaluates symbolic numbers in a more common way known as the Two’s Complement ; In this method, after the bits are inverted, the result is added to the number
1
. Therefore, in the example of the above code, the result of theNOT
number isa
equal11111100
, which is the representation of the number in base two in a complementary way:4 -
n = 3 0000 0011 3 = 0000 0011 (NOT) ----------- 1111 1100 = -3 (in One’s Complement) = -4 (in Two’s Complement)
Two’s Complement n = 4 0000 0100 4 = 0000 0100 (NOT) ----------- 1111 1011 1 ( + ) ----------- 1111 1100 = -4
The value can be considered equal to the product of the expression. Thus:
a ~
1 - a-
>>> ~ a + 1 -3
-
>>
Left Shift: Moves the left operand bits to the value of its right operand to the left – the rejected locations are set to zero:>>> a << 3 0000 0011 24 (LSH) ----------- 0001 1000 = 24
-
<<
Right Shift: Moves the left operand bits to the value of its right operand to the right – the rejected locations are set to zero:>>> a = 88 0101 1000 >>> a >> 3 (RSH) ----------- 11 0000 1011 = 11
-
=&
=|
=^
=>>
=<<
Combined operators (bit assignment): These operators first perform the bit operator operation on the operands on both sides and then attribute the result to the left operand.
5. Logical Operators:
These operators are not
or
and
used in conditional statements. Logical operators evaluate their operations based on True
(true) and False
(incorrect) values and return their results based on the table below. It is a not
single operand operator.
a | b | a and b | a or b | not a | not b |
---|---|---|---|---|---|
True | False | False | True | False | True |
False | True | False | True | True | False |
False | False | False | False | True | True |
True | True | True | True | False | False |
The operator returns and
only when both operands have a True value True
. The operator returns or
only when both operands have a False value False
. The operator not
also reverses its operating value (True to False and False to True).
6. Membership Operators:
Includes both operators in
and that they check for an amount among the members of a sequence (sequence such as strings, lists, etc.) are used.not in
in
: If the value of the left operand finds itself in the right operand ,True
andFalse
returns otherwise .not in
: If the value of the left operand does not find itself in the right operand ,True
otherwiseFalse
returns.
>>> "py" in "python"
True
>>> 1 in [1, 2, 3]
True
>>> "s" not in "python"
True
>>> 3 not in [1, 2, 3]
False
7. Identity Operators:
Includes both operator is
and that they are used to check one of the two objects.is not
is
If both operands reference to an object to be,True
and otherwiseFalse
returns.is not
: If both operands do not refer to the same object ,True
otherwiseFalse
returns.
>>> a = 3
>>> a is 3
True
Priorities
If a phrase contains multiple operators; Which action is examined first will have an impact on the outcome. Each of the operators has a priority that must be considered in order. At the bottom we will examine the priority of the operators – the priority is reduced from top to bottom:
() # Brackets ** ~ - + # Negative and positive * / // % - + # plus and minus << >> & ^ | ==! = <> < < => >= = **= /= // =% = *= -= += is is not in in not not and or
The parentheses have the highest priority, meaning that each phrase contained in it will have a higher priority for review; In nested parentheses, the innermost parentheses are the highest priority. If an expression contains several level operators; The operator’s priority is on the left. Note the following statements and their results:
>>> # Python 3.x >>> 4 + 2 - 3 + 2 * 5 13 >>> 4 + ((2 - 3) + 2) * 5 9 >>> 9 / 3 * 2 6.0 >>> 3 * 2 / 9 0.6666666666666666 >>> (5 - 3) ** (7 - 3) 16 >>> 4 + 3 ** 2 - 9 / 3 * 3 4.0 >>> 4 * 2 == 5 + 3 True